JavaScript meets Python: 10 Similarities, from a JavaScript Developer Perspective
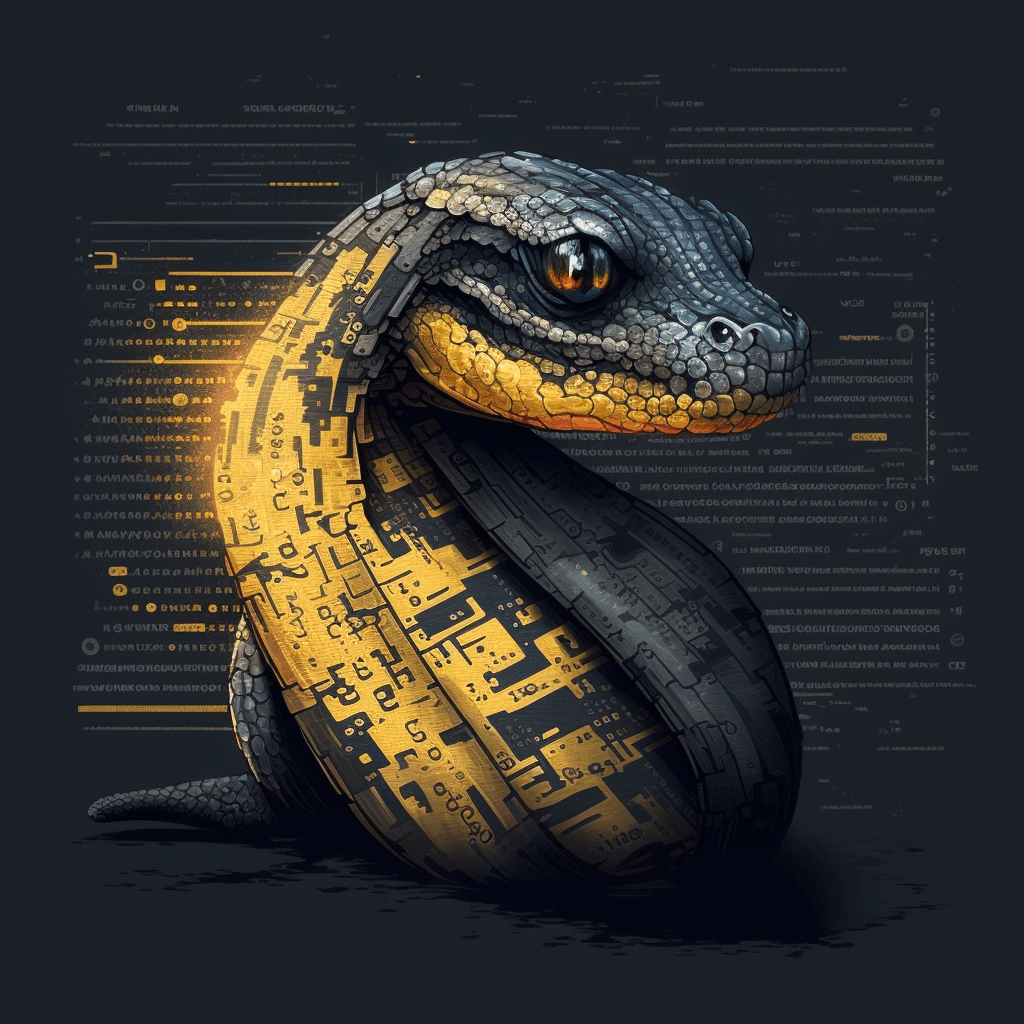
JavaScript and Python are two versatile programming languages that share interesting similarities.
One of the best ways to learn a new programming language is to compare it to a language you already know, so in this article, we explore 10 crossovers between these languages, showcasing their common or similar concepts and providing code examples.
Let's dive in! 🤿
1. Spread Operator (JavaScript) / Rest Operator (Python)
JavaScript Example:
const jediMasters = ["Obi-Wan", "Yoda", "Qui-Gon", "Mace Windu", "Anakin"];
const [firstJedi, secondJedi, ...padawans] = jediMasters;
console.log(padawans); // Output: "Qui-Gon", "Mace Windu", "Anakin"
Python Example:
jedi_masters = ["Obi-Wan", "Yoda", "Qui-Gon", "Mace Windu", "Anakin"]
first_jedi, second_jedi, *padawans = jedi_masters
print(padawans) # "Qui-Gon", "Mace Windu", "Anakin"
2. Ternary Operator (JavaScript) / Conditional Expression (Python)
JavaScript Example:
const distance = 7;
const travelMethod = distance > 10 ? "Car" : "Walk";
console.log(travelMethod); // Output: "Walk"
Python Example:
distance = 7
travel_method = "Car" if distance > 10 else "Walk"
print(travel_method) # Output: "Walk"
JavaScript Example:
const tossCoin = () => (Math.random() < 0.5 ? "Heads" : "Tails");
console.log(tossCoin()); // Output: 🪙🤞
Python Example:
import random
toss_coin = lambda: "Heads" if random.random() < 0.5 else "Tails"
print(toss_coin()) # Output: 🪙🤞
4. Object Destructuring (JavaScript) / Unpacking (Python)
JavaScript Example:
const character = {
name: "George Costanza",
age: 38,
catchphrase: "It's not a lie if you believe it!",
};
console.log(Object.values(character));
// Output: ['George Costanza', 38, "It's not a lie if you believe it!"]
Python Example:
character = {
'name': 'George Costanza',
'age': 38,
'catchphrase': "It's not a lie if you believe it!"
}
print(list(character.values()))
# Output: ['George Costanza', 38, "It's not a lie if you believe it!"]
5. Set Data Structure (JavaScript) / Python
JavaScript Example:
const superheroes = new Set([
"Batman",
"Superman",
"Wonder Woman",
"Spider-Man",
"Iron Man",
"Mysterio",
"Mysterio",
]);
console.log(superheroes); // Output: Set { 'Batman', 'Superman', 'Wonder Woman', 'Spider-Man', 'Iron Man', 'Mysterio' }
Python Example:
superheroes = {
"Batman",
"Superman",
"Wonder Woman",
"Spider-Man",
"Iron Man",
"Mysterio",
"Mysterio",
}
print(superheroes) # {'Batman', 'Superman', 'Wonder Woman', 'Spider-Man', 'Iron Man', 'Mysterio'}
6. String Interpolation, (JavaScript) / Python
JavaScript Example:
const petName = "Whiskers";
const petAge = 3;
console.log(`${petName} is ${petAge} years old.`);
// Output: 'Whiskers is 3 years old.'
Python Example:
pet_name = 'Whiskers'
pet_age = 3
print(f"{pet_name} is {pet_age} years old.")
# Output: 'Whiskers is 3 years old.'
7. Map Data Structure (JavaScript) / Python
JavaScript Example:
const animalSounds = new Map([
["Dog", "Bark"],
["Cat", "Meow"],
["Cow", "Moo"],
["Duck", "Quack"],
]);
console.log(animalSounds.get("Duck")); // Output: 'Quack'
Python Example:
animal_sounds = {
"Dog": "Bark",
"Cat": "Meow",
"Cow": "Moo",
"Duck": "Quack"
}
print(animal_sounds.get("Duck")) # Output: 'Quack'
8. Promises (JavaScript) / Futures (Python)
JavaScript Example:
const fetchPokemon = () => {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Pikachu caught successfully");
}, 3000);
});
};
const getPokemonMessage = async () => {
const data = await fetchPokemon();
return data;
};
getPokemonMessage().then((data) => console.log(data)); // Output: 'Pikachu caught successfully'
Python Example:
async def fetch_pokemon():
await asyncio.sleep(3)
return "Pikachu caught successfully"
async def get_pokemon_message():
data = await fetch_pokemon()
return data
print(asyncio.run(get_pokemon_message())) # 'Pikachu caught successfully'
9. Array Methods (map, filter, reduce) (JavaScript) / Python
JavaScript Example:
const numbers = [1, 2, 3, 4, 5];
// Using map()
const doubledNumbers = numbers.map((num) => num * 2);
console.log(doubledNumbers); // Output: [2, 4, 6, 8, 10]
// Using filter()
const evenNumbers = numbers.filter((num) => num % 2 === 0);
console.log(evenNumbers); // Output: [2, 4]
// Using reduce()
const sum = numbers.reduce(
(accumulator, currentValue) => accumulator + currentValue,
0
);
console.log(sum); // Output: 15
Python Example:
numbers = [1, 2, 3, 4, 5]
# Using map()
doubled_numbers = list(map(lambda num: num * 2, numbers))
print(doubled_numbers) # Output: [2, 4, 6, 8, 10]
# Using filter()
even_numbers = list(filter(lambda num: num % 2 == 0, numbers))
print(even_numbers) # Output: [2, 4]
# Using reduce()
from functools import reduce
sum = reduce(lambda x, y: x + y, numbers)
print(sum) # Output: 15
10. Modules and Imports (JavaScript) / Python
JavaScript Example:
// module.js
export function greet(name) {
console.log(`Hello, ${name}!`);
}
// main.js
import { greet } from "./module.js";
greet("Whiskers"); // Output: 'Hello, Whiskers!'
Python Example:
# module.py
def greet(name):
print(f'Hello, {name}!')
# main.py
from module import greet
greet('Whiskers') # Output: 'Hello, Whiskers!'
This is by all means not an exhaustive list of all the differences between JavaScript and Python. However, I hope it provided you with some fun analogies that help make information stick 🚀.